rfc1751.py: Python script to convert certificate fingerprint hash to ASCII words. Useful for distributing a given certificate fingerprint to VPN clients and SmartConsole users to avoid fingerprint warnings.
Examples:
./rfc1751.py 4607BC6AD16546324F12CD2D6EB43581
ASCII fingerprint string:
WOW SPA HIM JOKE BEAK HAT AUNT HELD ALOE SKAT USE MUSH
./rfc1751.py AB:3A:04:87:F0:83:07:DC:B6:22:45:0C:CC:8E:5D:B9:3F:FE:C3:4C
ASCII fingerprint string:
LAUD RISE KID SOAK OUR TORE MAIN EVA US HOBO SWAG SING
With inline "openssl s_client":
./rfc1751.py $(openssl s_client -connect 192.0.2.1:443 -showcerts </dev/null 2>/dev/null |sed -n -e '/0 s:/,/END CERT/p'|openssl x509 -noout -fingerprint -sha1|awk -F= '{print $2}')
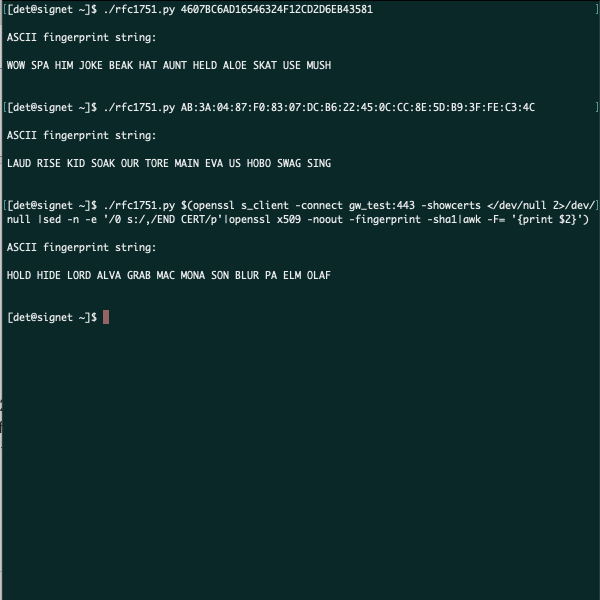
Notes:
If you are using the Windows certificate details view, the Thumbprint value is 40-bytes, long not 32, so that has to be truncated (which the script does). Feel free to paste the whole 40-byte string as the argument. If you are using openssl x509 -fingerprint, then use the -sha1 argument to get the SHA1 hash (may be default, but a newer openssl may change that some day).
#!/usr/bin/python3
import sys, os
import binascii
from Crypto.Util import RFC1751
def main(argv=None):
if argv is None:
argv = sys.argv
if not (len(argv)):
print("Fingerprint hex string missing\n")
print("Usage: {} <string>\n".format(__file__))
print("String may be colon-separated hex (00:01:aa:bb...) or without colons (0001abcd...)\n")
exit(1)
fingerprint_arg=argv[0]
fingerprint_str=fingerprint_arg.replace(':','')
if (len(fingerprint_str) == 40):
fingerprint_hex=fingerprint_str[:32]
elif (len(fingerprint_str) == 32):
fingerprint_hex=fingerprint_str
else:
print("Usage: {} <string>\n".format(__file__))
exit(1)
fingerprint_txt=RFC1751.key_to_english(binascii.unhexlify(fingerprint_hex[:32]))
print("\nASCII fingerprint string:\n")
print(fingerprint_txt)
print("\n")
if __name__ == "__main__":
main(sys.argv[1:])