Hello. I wrote python script to get result of cpstat fw command:
def api_call(ip_addr, port, command, json_payload, sid):
url = 'https://' + ip_addr + ':' + str(port) + '/web_api/' + command
if sid == '':
request_headers = {'Content-Type' : 'application/json'}
else:
request_headers = {'Content-Type' : 'application/json', 'X-chkp-sid' : sid}
r = requests.post(url,data=json.dumps(json_payload), headers=request_headers, verify=False)
return r.json()
def login(user,password):
payload = {'user':user, 'password' : password}
response = api_call(mgmt_server, 443, 'login',payload, '')
return response["sid"]
def run_script(script_name, script_command,sid):
payload = {'script-name':script_name, 'script':script_command, 'targets':otr_gws}
response = api_call(mgmt_server, 443, 'run-script', payload, sid)
return response
def show_task(task_id, sid):
payload = {'task-id':task_id, 'details-level':'full'}
response = api_call(mgmt_server, 443, 'show-task', payload, sid)
return response
def main():
sid = login(mgmt_username,mgmt_password)
print("session id: " + sid)
cpstat = run_script("cpstat","cpstat fw",sid)
print(cpstat)
print("------------------------------")
print("cpstat result: " + json.dumps(cpstat))
print("------------------------------")
task_id=cpstat["tasks"][0]["task-id"]
result=show_task(task_id, sid)
print(result)
logout_result = api_call(mgmt_server, 443,"logout", {},sid)
print("logout result: " + json.dumps(logout_result))
if __name__ == '__main__':
main()
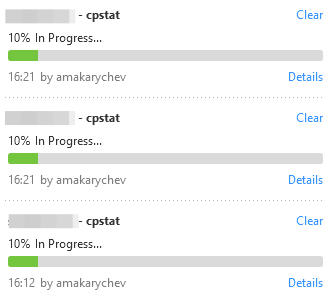
But when I run script it just stucks on the management server and nothing hashappened. What could be the problem?
P.S. The main idea is I want to make script to check ISP Redundancy status and to switch ISP Manually.